- 下载源160.2 KB
您可以在此处查看此项目的源代码和最新更新
这是Angular/.NET Core Web API入门应用程序,具有添加、编辑和删除客户的基本功能,因此您可以将其用作构建应用程序的起点。它使用以下框架:
- Angular Material
- Bootstrap
- .NET Core
- Entity Frameworks
如何在电脑上运行
请确保首先安装以下组件:
- 节点v12.19。*
- Angular CLI 10.2。*
- .NET Core 3.1
在下载的代码中,打开文件api/Api/appsettings.Development.json并将数据源更改为指向您的SQL Server:
"AngApiStarterConn": "Data Source=YourServerNameHere;
Initial Catalog=AngApiStarter;Integrated Security=True;"
生成API解决方案以确保已安装NuGet软件包,并且该解决方案成功生成。您必须先成功构建解决方案,然后才能为数据库运行Entity Framework更新。
打开命令提示符并导航到api/Data,然后运行以下命令,该命令将使用应用程序所需的结构更新SQL Server:
dotnet ef database update -s ..\Api\Api.csproj
您现在应该拥有一个名为AngApiStarter的数据库,该数据库具有一个在SQL Server中命名为Customers的表。您可以将带有下面的SQL的示例插入customer表中:
insert into customers(firstName, lastName)
values('John', 'Smith');
通过运行API项目并检查以下URL,检查API是否可以从数据库获取数据:URL应该返回数据库中的customers列表:
https://localhost:44381/api/customer
导航至命令提示符下的ui文件夹,然后运行以下命令以获取运行Angular所需的库:
npm update
如果遇到找不到模块的错误,则可以运行以下命令以获取所需的模块:
npm install
通过运行以下命令来启动Angular,它将启动服务器并在浏览器中打开应用程序:
ng serve --open
应用程序的构建方式
从API的数据层开始,创建了Customer的类模型:
public class Customer
{
[Required]
public int CustomerId { get; set; }
[Required, StringLength(80)]
public string FirstName { get; set; }
[Required, StringLength(80)]
public string LastName { get; set; }
}
然后为Customer类创建DbContext,使用Customer类作为DbSet,其最终将成为数据库中的表:
public class AngApiStarterDbContext : DbContext
{
public DbSet Customer { get; set; }
public AngApiStarterDbContext(DbContextOptions options)
: base(options)
{
}
}
然后,在API项目中,我们定义数据库连接字符串,并将以下代码添加到Startup.cs的ConfigureServices方法中,如下所示。这样我们就可以使用API项目中定义的连接字符串在SQL Server中创建数据库和表:
//add the db context with the connection string
services.AddDbContextPool(options =>
options.UseSqlServer(Configuration.GetConnectionString("AngApiStarterConn"))
);
生成解决方案,然后使用命令提示符,导航到Data项目文件夹,然后运行以下命令以创建数据库迁移的类:
dotnet ef migrations add initialCreate -s ..\Api\Api.csproj
创建类之后,运行以下命令以在SQL Server中创建数据库和表:
dotnet ef database update -s ..\Api\Api.csproj
在Data项目中,它具有接口以及使用实体框架的接口实现:
public interface ICustomerData
{
Task Get();
Task GetCustomerById(int id);
Task Update(Customer customer);
Task Add(Customer customer);
Task Delete(int customerId);
}
public class SqlCustomerData : ICustomerData
{
public AngApiStarterDbContext DbContext { get; }
public SqlCustomerData(AngApiStarterDbContext dbContext)
{
DbContext = dbContext;
}
...
}
现在进入API项目。在API项目中,它在ConfigureServices方法中定义接口的实现类:
services.AddScoped();
然后,控制器也通过依赖注入使用该接口:
public class CustomerController : ControllerBase
{
public ICustomerData CustomerData { get; }
public CustomerController(ICustomerData customerData)
{
CustomerData = customerData;
}
...
由于API和UI将在不同的网站上运行,因此需要允许UI网站使用CORS访问API。在API的appsettings.Development.json中,它具有UI的url:
"CorsUrl": "http://localhost:4200"
然后在Startup.cs文件中指定CORS策略:
private readonly string corsPolicy = "defaultPolicy";
public void ConfigureServices(IServiceCollection services)
{
services.AddCors(options =>
{
options.AddPolicy(corsPolicy,
builder =>
{
builder.WithOrigins(Configuration["CorsUrl"])
.AllowAnyMethod()
.AllowAnyHeader()
.AllowCredentials();
});
});
...
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
...
app.UseCors(corsPolicy);
...
}
现在进入Angular的UI项目。使用Angular入门项目,在项目app/common/material.module.ts下定义了Angular Materials组件,在其中添加了所有组件,以便您可以使用这些组件而无需将其显式添加到app.module.ts。然后在app.module.ts中,它仅包含Material模块:
import { MaterialModule } from './common/material.module';
API URL的位置在environment/environments.ts文件中定义:
export const environment = {
production: false,
apiCustomer: 'https://localhost:44381/api/customer'
};
该模型在model/ICustomer.ts文件中定义,具有与API相同的模型签名:
export interface ICustomer{
customerId: number,
firstName: string,
lastName: string
}
与API交互的支持位于service/customer.service.ts文件中。它使用BehaviorSubject将客户列表作为Observable(可以将其视为数据流)。当Observable改变时,所有的观察员(你可以把它当作听众或用户)得到通知的变化,可以相应地做出反应。在CustomerService类中,需要在Observable需要通知所有观察者时调用customerList.next方法:
private customerList = new BehaviorSubject(null);
customerList$ = this.customerList.asObservable();
...
//get the list of customers
get(){
this.http.get(this.url).pipe(
).subscribe(result => {
if (result)
//notify the Observers of the change
this.customerList.next(result)
});
}
Customer组件显示了您可以管理的客户列表,它通过在ngOnInit方法中订阅CustomerService类来监听类中的更改:
export class CustomerComponent implements OnInit {
...
ngOnInit(){
this.custService.customerList$.subscribe(data =>
this.customerList = data
);
}
当用户单击“添加”或“编辑客户”按钮时,它将打开一个对话框,您可以将数据传递给该对话框,并定义关闭该对话框时要执行的操作。下面显示了添加一个新的customer并在关闭对话框时调用CustomerService时传递给该对话框的数据:
openAddDialog(){
let dialogRef = this.custDialog.open(CustomerDialog, {
width: '300px',
data: {
action: 'add',
customer: { customerId: 0, firstName: '', lastName: ''}
}
});
dialogRef.afterClosed().subscribe(result => {
//add the customer
if (result)
this.custService.add(result)
});
}
为了进行编辑customer,它需要将customer数据的副本而不是实际的customer数据传递给对话框,因此,如果用户决定取消,则customer值不会更改:
customer: {...customer} //shallow copy the customer using spread operator
对话框组件是在app/customer/dialog/customer-dialog.component.ts中定义的,该组件使来自Material的Dialog组件的MAT_DIALOG_DATA接受调用者的数据:
export class CustomerDialog {
...
constructor(public dialogRef: MatDialogRef,
@Inject(MAT_DIALOG_DATA) public data: any) {
this.action = data.action;
this.customer = data.customer;
}
最后,使用npm添加bootstrap库,并将对bootstrap样式表的引用添加至index.html:
关注
打赏
最近更新
- 深拷贝和浅拷贝的区别(重点)
- 【Vue】走进Vue框架世界
- 【云服务器】项目部署—搭建网站—vue电商后台管理系统
- 【React介绍】 一文带你深入React
- 【React】React组件实例的三大属性之state,props,refs(你学废了吗)
- 【脚手架VueCLI】从零开始,创建一个VUE项目
- 【React】深入理解React组件生命周期----图文详解(含代码)
- 【React】DOM的Diffing算法是什么?以及DOM中key的作用----经典面试题
- 【React】1_使用React脚手架创建项目步骤--------详解(含项目结构说明)
- 【React】2_如何使用react脚手架写一个简单的页面?

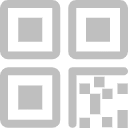
微信扫码登录