- 准备工程,在此链接的例程上增加SYSTICK功能
https://blog.csdn.net/mygod2008ok/article/details/106748615
- 配置系统时钟
/* Private function prototypes -----------------------------------------------*/
/**
* @brief System Clock Configuration
* The system Clock is configured as follow :
* System Clock source = PLL (HSI/2)
* SYSCLK(Hz) = 48000000
* HCLK(Hz) = 48000000
* AHB Prescaler = 1
* APB1 Prescaler = 1
* HSI Frequency(Hz) = 8000000
* PREDIV = 1
* PLLMUL = 12
* Flash Latency(WS) = 1
* @param None
* @retval None
*/
static void SystemClock_Config(void)
{
RCC_ClkInitTypeDef RCC_ClkInitStruct;
RCC_OscInitTypeDef RCC_OscInitStruct;
/* No HSE Oscillator on Nucleo, Activate PLL with HSI/2 as source */
RCC_OscInitStruct.OscillatorType = RCC_OSCILLATORTYPE_LSI;
RCC_OscInitStruct.PLL.PLLState = RCC_PLL_ON;
RCC_OscInitStruct.PLL.PLLSource = RCC_PLLSOURCE_HSI;
RCC_OscInitStruct.LSIState = RCC_LSI_ON;
RCC_OscInitStruct.HSICalibrationValue = 16;
RCC_OscInitStruct.PLL.PREDIV = RCC_PREDIV_DIV1;
RCC_OscInitStruct.PLL.PLLMUL = RCC_PLL_MUL12;
HAL_StatusTypeDef err_code = HAL_RCC_OscConfig(&RCC_OscInitStruct);
APP_ERROR_CHECK(err_code);
/* Select PLL as system clock source and configure the HCLK, PCLK1 clocks dividers */
RCC_ClkInitStruct.ClockType = (RCC_CLOCKTYPE_SYSCLK | RCC_CLOCKTYPE_HCLK | RCC_CLOCKTYPE_PCLK1);
RCC_ClkInitStruct.SYSCLKSource = RCC_SYSCLKSOURCE_PLLCLK;
RCC_ClkInitStruct.AHBCLKDivider = RCC_SYSCLK_DIV1;
RCC_ClkInitStruct.APB1CLKDivider = RCC_HCLK_DIV1;
err_code = HAL_RCC_ClockConfig(&RCC_ClkInitStruct, FLASH_LATENCY_1);
APP_ERROR_CHECK(err_code);
}
- 在sdk_config.h中加入SYS_TICK_COUNTER宏,这里将SYSTICK配置成25Hz
#ifndef SDK_CONFIG_H
#define SDK_CONFIG_H
#define STM_MODULE_ENABLED(module) \
((defined(module ## _ENABLED) && (module ## _ENABLED)) ? 1 : 0)
// >\n
//==================================================================================================
// 系统嘀嗒配置
// 系统嘀嗒时间
#ifndef SYS_TICK_COUNTER
#define SYS_TICK_COUNTER 25
#endif
//
//==========================================================
// DEBUG配置
// DEBUG使能
#ifndef DEBUG_ENABLED
#define DEBUG_ENABLED 1
#endif
// ASSERT参数检查使能
#ifndef IS_USE_FULL_ASSERT
#define IS_USE_FULL_ASSERT 1
#endif
#if IS_USE_FULL_ASSERT
#define USE_FULL_ASSERT
#endif
//
// >
#endif //SDK_CONFIG_H
- 重写__weak弱编译HAL_InitTick函数,这样就将SYSTICK频率配置成SYS_TICK_COUNTER,即25Hz,也就是每个tick时间为40毫秒
/**
* @brief overload system tick
*
*/
HAL_StatusTypeDef HAL_InitTick(uint32_t TickPriority)
{
/*Configure the SysTick to have interrupt in 1ms time basis*/
HAL_SYSTICK_Config(HAL_RCC_GetHCLKFreq()/SYS_TICK_COUNTER);
/*Configure the SysTick IRQ priority */
HAL_NVIC_SetPriority(SysTick_IRQn, TickPriority ,0U);
/* Return function status */
return HAL_OK;
}
- 在main.C文件中定义变量
volatile uint16_t s_wakeup_flag; // 唤醒事件标志
- 在main.h中声明变量及定义事件标志宏
/* USER CODE BEGIN Header */
/**
******************************************************************************
* @file : main.h
* @brief : Header for main.c file.
* This file contains the common defines of the application.
******************************************************************************
* @attention
*
* © Copyright (c) 2020 STMicroelectronics.
* All rights reserved.
*
* This software component is licensed by ST under BSD 3-Clause license,
* the "License"; You may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
* opensource.org/licenses/BSD-3-Clause
*
******************************************************************************
*/
/* USER CODE END Header */
/* Define to prevent recursive inclusion -------------------------------------*/
#ifndef __MAIN_H
#define __MAIN_H
#ifdef __cplusplus
extern "C" {
#endif
/* Includes ------------------------------------------------------------------*/
#include "stm32f0xx_hal.h"
#define TICK_FOR_25HZ 0x02 //!< 40ms事件标志
#define CLEAR_TICK_FOR_25HZ (~TICK_FOR_25HZ) //!< 清除40ms事件标志
extern volatile uint16_t s_wakeup_flag;
#ifdef __cplusplus
}
#endif
#endif /* __MAIN_H */
/************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
- 在stm32f0xx_it.c中包含头文件,并在SysTick_Handler中断处理函数中加入以下代码
/**
* @brief This function handles System tick timer.
*/
void SysTick_Handler(void)
{
/* USER CODE BEGIN SysTick_IRQn 0 */
/* USER CODE END SysTick_IRQn 0 */
HAL_IncTick();
/* USER CODE BEGIN SysTick_IRQn 1 */
s_wakeup_flag |= TICK_FOR_25HZ;
/* USER CODE END SysTick_IRQn 1 */
}
- 在main.c中实现25Hz处理函数
/**
* @brief 25Hz handler
*
*/
void tick_25hz_handler(void)
{
if(s_wakeup_flag & TICK_FOR_25HZ)
{
s_wakeup_flag &= CLEAR_TICK_FOR_25HZ;
//####################################################################################
//---------TODO this to add 25hz event handler-----------
NRF_LOG_INFO("tick_25hz_and_1hz_handler");
}
}
- 在循环中调用tick_25hz_handler函数
/* USER CODE END 0 */
/**
* @brief The application entry point.
* @retval int
*/
int main(void)
{
/* USER CODE BEGIN 1 */
RTT_INIT();
HAL_Init();
SystemClock_Config();
NRF_LOG_INFO("program is reset!");
BSP_wdt_init(5000);
/* Infinite loop */
/* USER CODE BEGIN WHILE */
while (1)
{
/* USER CODE END WHILE */
BSP_wdt_feed();
tick_25hz_handler();
/* USER CODE BEGIN 3 */
}
/* USER CODE END 3 */
}
- 运行结果如下:
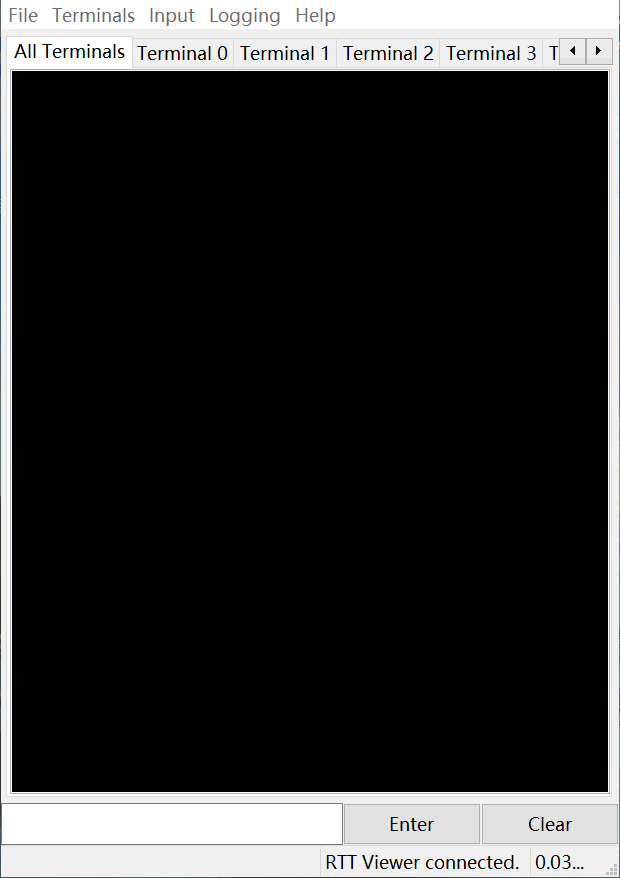
https://download.csdn.net/download/mygod2008ok/12522570