一、jquery标签选择器的使用
获取id为example的div用$('#example')
$("#a1").css("background-color","red"); // 根据id
根据class类别
$(".c1").css("background-color","red"); // 根据class类别
根据标签元素
$("a").css("background-color","red");
$("div").css("color","yellow");
$("button").click(function(){
$("li").each(function(){
alert($(this).text())
});
});
$('td[aria="View_CHECK_NAME"]').each(function(){
if($(this).html()=="yes"){
$(this).attr("style","color:red; text-align:center;cursor:pointer");
}
})
根据属性
$("[href]").css("background-color","red");
$("[href='#']").css("background-color","red");
$("[href$='com']").css("background-color","red");
$("div[class=logo]").children("img").attr("src");
$("button[name='comQue']").each(function(index,item){
$(this).trigger("click");
)}
获取标签元素的属性
$("img").attr("src"); //返回文档中所有图像的src属性值
$("img").attr("src","test.jpg"); //设置所有图像的src属性
$("img").removeAttr("src"); //将文档中图像的src属性删除
$(document).ready(function(){
var headPicture = $("#headPicture");
if(headPicture&&headPicture.length>0){
var imgSrc = $($(headPicture)[0]).attr("src");
$("div[class=logo]").children("img").attr("src",imgSrc);
}
})
if($(this).html()=="yes"){
$(this).attr("style","color:red; text-align:center;cursor:pointer");
}
Attr用法一:设置单个值
$(selector).attr(attribute,value)
$("img").attr("width","180");
Attr用法二:设置多个值
$(selector).attr({attribute:value, attribute:value ...})
$("img").attr({width:"50",height:"80"});
Attr用法三:使用函数设置属性
$(selector).attr(attribute,function(index,oldvalue))
index:选择器的 index 值
oldvalue:当前属性值
$("img").attr("width",function(n,v){
return v-50;
});
removeAttr
$(selector).removeAttr(attribute)
$("p").removeAttr("style");
获取标签样式
$("p").css("color"); //访问查看p元素的color属性
$("p").css("color","red"); //设置p元素的color属性为red
$("#a1").css("background-color","red");
$("div").css("height","30px");
$("p").css({ "color": "red", "background": "yellow" }); //设置p元素的color为red,background属性为yellow(设置多个属性要用{}字典形式)
$("p").addClass("selected"); //为p元素加上 'selected' 类
$("p").removeClass("selected"); //从p元素中删除 'selected' 类
$("p").toggleClass("selected"); //如果存在就删除,否则就添加
$("p.c1").css("background-color","red");
$("ul li:first").css("background-color","red");
$("ul li:last").css("background-color","red");
根据标签位置与内容来获取
$('li:first') //第一个元素
$('li:last') //最后一个元素
$('li').first() //第一个元素
$('li').last() //最后一个元素
$("tr:even") //索引为偶数的元素,从 0 开始
$("tr:odd") //索引为奇数的元素,从 0 开始
$("tr:eq(1)") //给定索引值的元素
$("p").eq(0) //当前操作中第N个jQuery对象,类似索引
$("tr:gt(0)") //大于给定索引值的元素
$("tr:lt(2)") //小于给定索引值的元素
$(":focus") //当前获取焦点的元素
$(":animated") //正在执行动画效果的元素
$(":text").val(""); //这个能把input是text类型的全部重置了
$(":input") //匹配所有 input, textarea, select 和 button 元素
$(":text") //所有的单行文本框
$(":password") //所有密码框
$(":radio") //所有单选按钮
$(":checkbox") //所有复选框
$(":submit") //所有提交按钮
$(":reset") //所有重置按钮
$(":button") //所有button按钮
$(":file") //所有文件域
$("#box_list1 tr:not(:first)").empty("");//根据id把不是第一个的全部置空
$("td:empty") //不包含子元素或者文本的空元素
$("div:contains('nick')") //包含nick文本的元素
$("div:has(p)") //含有选择器所匹配的元素
$(this).hasClass("test") //元素是否含有某个特定的类,返回布尔值
$('li').has('ul') //包含特定后代的元素
$("input:checked") //所有选中的元素
$("select option:selected") //select中所有选中的option元素
$("input[type='checkbox']").prop("checked", true); //选中复选框
$("input[type='checkbox']").prop("checked", false);
$("img").removeProp("src"); //删除img的src属性
标签相互嵌套的规则获取
$("p.c1").css("background-color","red");
$("ul li:first").css("background-color","red");
$("ul li:last").css("background-color","red");
div2内的文本
span内有文本内容
console.log($("#div2 span").html());
$(".layui-input-block $(".layui-input-block button[id='subjectSmallImage']").off('click',a).on('click',upload);
可以同时绑定多个标签
$("ul li,div").on("click",function(){
alert("不响应事件!");
})
根据某一范围内来确定层次关系,比如在trs[index+1]范围内的td:eq(1)的标签。在后面范围内的查找前面的元素
$("input:first",$("td:eq(1)",$(trs[index+1]))).attr("checked",true);//第一个选中
根据层级来获取
$("ul>li>input:checked").each(function(i,el){
$(this).prop("checked",true);
});
其他
$('p').offset() //元素在当前视口的相对偏移,Object {top: 122, left: 260}
$('p').offset().top
$('p').offset().left
$("p").position() //元素相对父元素的偏移,对可见元素有效,Object {top: 117, left: 250}
$(window).scrollTop() //获取滚轮滑的高度
$(window).scrollLeft() //获取滚轮滑的宽度
$(window).scrollTop('100') //设置滚轮滑的高度为100
$("p").height(); //获取p元素的高度
$("p").width(); //获取p元素的宽度
$("p:first").innerHeight() //获取第一个匹配元素内部区域高度(包括补白、不包括边框)
$("p:first").innerWidth() //获取第一个匹配元素内部区域宽度(包括补白、不包括边框)
$("p:first").outerHeight() //匹配元素外部高度(默认包括补白和边框)
$("p:first").outerWidth() //匹配元素外部宽度(默认包括补白和边框)
$("p:first").outerHeight(true) //为true时包括边距
jquery动态生成页签
- 基本信息
- 商业
- 农业
- 工业
- 税收
- 财政
- 营收
日期
值班员
部门
计划
实际
月计划量
年计划量
全部
js
$(function(){
// $('.tb_table table input').attr("disabled","false");
});
// tab切换
$(function(){
function switchTab(liDe, divDe,elentDe,classDe,timeDe){
liDe.each(function(i){
var liDeThis = $(this);
liDeThis.bind(elentDe,function(){
liDe.removeClass();
divDe.stop().hide().animate({'opacity':0},0);
$(this).addClass(classDe);
divDe.eq(i).show().animate({'opacity':1},timeDe);
});
});
}
switchTab($(".tb_tab").find(".tb_col li"), $(".tb_tab").find(".turn"),"click","on",300);
});
获取选中的页签
var u =$(".tb_tab .tb_col ul").children(); //通过选择器获取页签
for(var i =0;i
关注
打赏
最近更新
- 深拷贝和浅拷贝的区别(重点)
- 【Vue】走进Vue框架世界
- 【云服务器】项目部署—搭建网站—vue电商后台管理系统
- 【React介绍】 一文带你深入React
- 【React】React组件实例的三大属性之state,props,refs(你学废了吗)
- 【脚手架VueCLI】从零开始,创建一个VUE项目
- 【React】深入理解React组件生命周期----图文详解(含代码)
- 【React】DOM的Diffing算法是什么?以及DOM中key的作用----经典面试题
- 【React】1_使用React脚手架创建项目步骤--------详解(含项目结构说明)
- 【React】2_如何使用react脚手架写一个简单的页面?
立即登录/注册

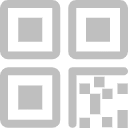
微信扫码登录